Building Scalable Django Applications
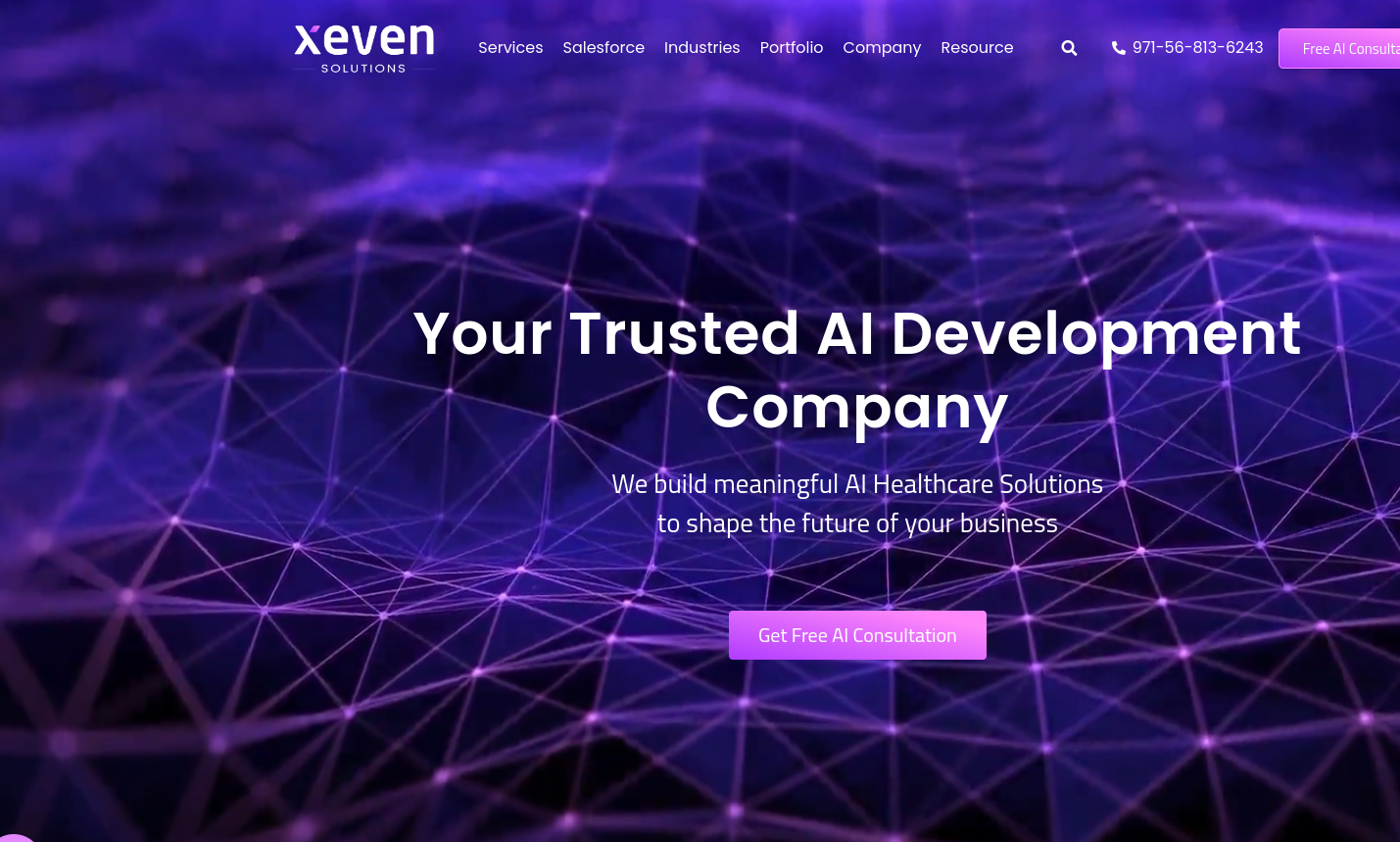
# Building Scalable Django Applications
When developing web applications that need to handle significant traffic and data, scalability becomes a critical concern. Django provides several features and best practices that can help you build applications that scale effectively.
## Database Optimization
One of the most important aspects of scalability is database optimization. Django's ORM provides several tools to help you optimize database queries:
- Use `select_related()` and `prefetch_related()` to reduce the number of queries
- Implement database indexes for frequently queried fields
- Use `django-debug-toolbar` to identify and fix N+1 query problems
- Consider using database connection pooling
## Caching Strategies
Implementing a proper caching strategy can significantly improve performance:
- Use Django's cache framework with Redis or Memcached
- Implement view-level caching for expensive operations
- Consider template fragment caching for reusable components
- Use the cache_page decorator for static content
## Background Task Processing
Moving time-consuming tasks to background workers can improve response times:
- Use Celery for asynchronous task processing
- Implement task queues for email sending, file processing, etc.
- Consider using Django Channels for real-time features
## Horizontal Scaling
Design your application to scale horizontally from the beginning:
- Ensure your application is stateless
- Use load balancers to distribute traffic
- Store sessions in a centralized cache or database
- Consider microservices architecture for complex applications
By following these best practices, you can build Django applications that scale effectively to handle growing user demands and traffic.