Securing Django Applications
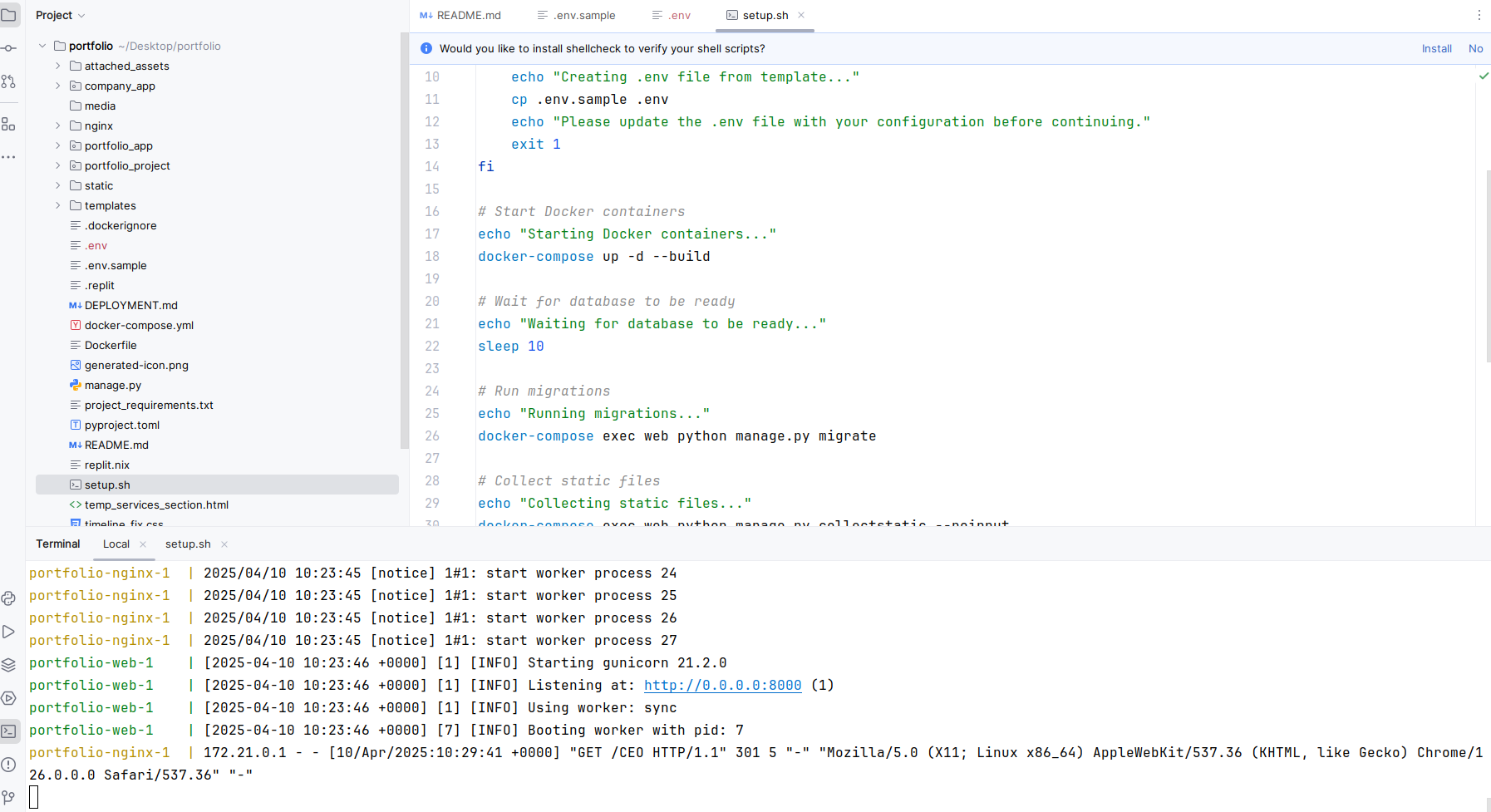
# Securing Django Applications
Security is a critical aspect of web application development. Django provides many built-in security features, but it's important to understand and implement additional measures to ensure your application is as secure as possible.
## HTTPS Implementation
Always use HTTPS in production environments:
- Configure SSL/TLS certificates properly
- Use SECURE_SSL_REDIRECT setting to force HTTPS
- Set SECURE_HSTS_SECONDS to enable HTTP Strict Transport Security
- Configure secure cookies with SESSION_COOKIE_SECURE and CSRF_COOKIE_SECURE
## Cross-Site Scripting (XSS) Prevention
Protect your application from XSS attacks:
- Django's template system automatically escapes HTML, but be cautious with safe filter
- Use Content Security Policy (CSP) headers
- Validate and sanitize user input
- Be cautious when including user-generated content
## CSRF Protection
Cross-Site Request Forgery protection is essential:
- Always use Django's CSRF protection middleware
- Include {% csrf_token %} in all forms
- Implement proper CSRF handling for AJAX requests
## Authentication Security
Secure your authentication system:
- Implement password policies (complexity, expiration, etc.)
- Use Django's built-in password hashers
- Consider two-factor authentication for sensitive applications
- Implement account lockout after failed login attempts
## SQL Injection Prevention
Django's ORM helps prevent SQL injection, but be careful with raw queries:
- Avoid using raw SQL when possible
- Use parameterized queries when raw SQL is necessary
- Never use string formatting to build SQL queries
## Security Headers
Implement security headers to enhance protection:
- X-Content-Type-Options: nosniff
- X-Frame-Options: DENY or SAMEORIGIN
- X-XSS-Protection: 1; mode=block
- Content-Security-Policy
By implementing these security measures, you can significantly reduce the risk of security vulnerabilities in your Django applications.